对于最新的稳定版本,请使用 Spring Security 6.5.0! |
使用 AuthorizationFilter 授权 HttpServletRequests
本节以 Servlet 体系结构和实现为基础,深入探讨了授权在基于 Servlet 的应用程序中的工作原理。
AuthorizationFilter 取代FilterSecurityInterceptor .
为了保持向后兼容,FilterSecurityInterceptor 仍然是默认值。
本节讨论如何AuthorizationFilter 有效以及如何覆盖默认配置。 |
这AuthorizationFilter
提供HttpServletRequest
s.
它作为 Security Filters 之一插入到 FilterChainProxy 中。
您可以在声明SecurityFilterChain
.
而不是使用authorizeRequests
用authorizeHttpRequests
这样:
-
Java
@Bean
SecurityFilterChain web(HttpSecurity http) throws AuthenticationException {
http
.authorizeHttpRequests((authorize) -> authorize
.anyRequest().authenticated();
)
// ...
return http.build();
}
这改进了authorizeRequests
以多种方式:
-
使用简化的
AuthorizationManager
API 而不是元数据源、配置属性、决策管理器和投票者。 这简化了重用和定制。 -
延误
Authentication
查找。 它不需要为每个请求查找身份验证,而只会在授权决策需要身份验证的请求中查找身份验证。 -
基于 Bean 的配置支持。
什么时候authorizeHttpRequests
替换为authorizeRequests
然后AuthorizationFilter
替换为FilterSecurityInterceptor
.
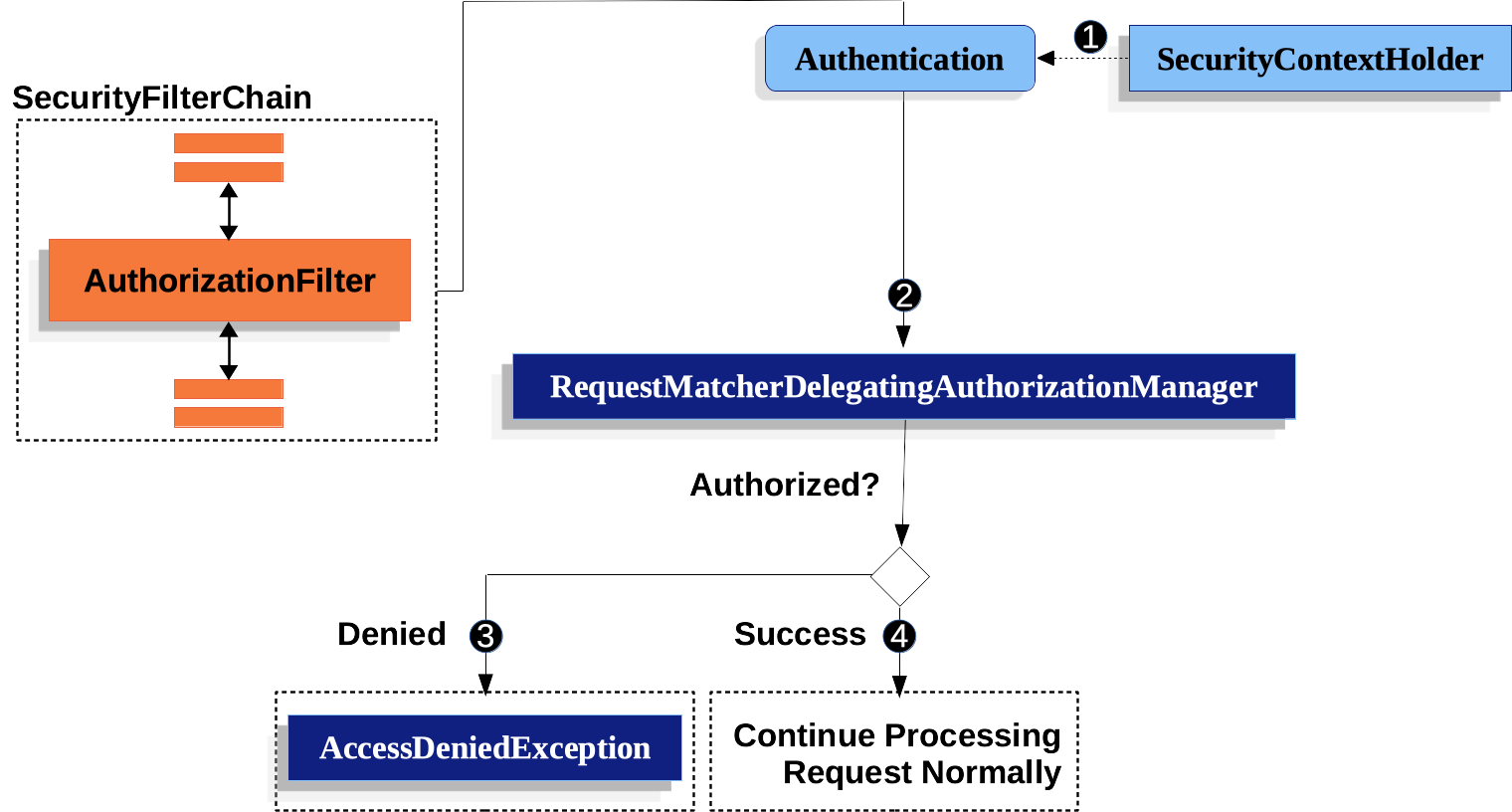
-
首先,
AuthorizationFilter
从SecurityContextHolder获取Authentication。 它将其包装在Supplier
以延迟查找。 -
其次,它将
Supplier<Authentication>
和HttpServletRequest
到AuthorizationManager
.-
如果授权被拒绝,则
AccessDeniedException
被抛出。 在这种情况下,ExceptionTranslationFilter
处理AccessDeniedException
. -
如果授予访问权限,
AuthorizationFilter
继续使用 FilterChain,它允许应用程序正常处理。
-
我们可以通过按优先顺序添加更多规则来将 Spring Security 配置为具有不同的规则。
-
Java
@Bean
SecurityFilterChain web(HttpSecurity http) throws Exception {
http
// ...
.authorizeHttpRequests(authorize -> authorize (1)
.mvcMatchers("/resources/**", "/signup", "/about").permitAll() (2)
.mvcMatchers("/admin/**").hasRole("ADMIN") (3)
.mvcMatchers("/db/**").access((authentication, request) ->
Optional.of(hasRole("ADMIN").check(authentication, request))
.filter((decision) -> !decision.isGranted())
.orElseGet(() -> hasRole("DBA").check(authentication, request));
) (4)
.anyRequest().denyAll() (5)
);
return http.build();
}
1 | 指定了多个授权规则。 每个规则都按照其声明的顺序进行考虑。 |
2 | 我们指定了任何用户都可以访问的多个 URL 模式。 具体而言,如果 URL 以“/resources/”开头、等于“/signup”或等于“/about”,则任何用户都可以访问请求。 |
3 | 任何以 “/admin/” 开头的 URL 都将被限制为具有 “ROLE_ADMIN” 角色的用户。
您会注意到,由于我们正在调用hasRole 方法,则不需要指定 “ROLE_” 前缀。 |
4 | 任何以 “/db/” 开头的 URL 都要求用户同时具有 “ROLE_ADMIN” 和 “ROLE_DBA”。
您会注意到,由于我们使用的是hasRole 表达式,则不需要指定 “ROLE_” 前缀。 |
5 | 任何尚未匹配的 URL 都将被拒绝访问。 如果您不想意外忘记更新授权规则,这是一个很好的策略。 |
您可以通过构建自己的方法来采用基于 bean 的方法RequestMatcherDelegatingAuthorizationManager
这样:
-
Java
@Bean
SecurityFilterChain web(HttpSecurity http, AuthorizationManager<RequestAuthorizationContext> access)
throws AuthenticationException {
http
.authorizeHttpRequests((authorize) -> authorize
.anyRequest().access(access)
)
// ...
return http.build();
}
@Bean
AuthorizationManager<RequestAuthorizationContext> requestMatcherAuthorizationManager(HandlerMappingIntrospector introspector) {
RequestMatcher permitAll =
new AndRequestMatcher(
new MvcRequestMatcher(introspector, "/resources/**"),
new MvcRequestMatcher(introspector, "/signup"),
new MvcRequestMatcher(introspector, "/about"));
RequestMatcher admin = new MvcRequestMatcher(introspector, "/admin/**");
RequestMatcher db = new MvcRequestMatcher(introspector, "/db/**");
RequestMatcher any = AnyRequestMatcher.INSTANCE;
AuthorizationManager<HttpRequestServlet> manager = RequestMatcherDelegatingAuthorizationManager.builder()
.add(permitAll, (context) -> new AuthorizationDecision(true))
.add(admin, AuthorityAuthorizationManager.hasRole("ADMIN"))
.add(db, AuthorityAuthorizationManager.hasRole("DBA"))
.add(any, new AuthenticatedAuthorizationManager())
.build();
return (context) -> manager.check(context.getRequest());
}
您还可以为任何请求匹配器连接自己的自定义授权管理器。
以下是将自定义授权管理器映射到my/authorized/endpoint
:
-
Java
@Bean
SecurityFilterChain web(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests((authorize) -> authorize
.mvcMatchers("/my/authorized/endpoint").access(new CustomAuthorizationManager());
)
// ...
return http.build();
}
或者你可以为所有请求提供它,如下所示:
-
Java
@Bean
SecurityFilterChain web(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests((authorize) -> authorize
.anyRequest.access(new CustomAuthorizationManager());
)
// ...
return http.build();
}
默认情况下,AuthorizationFilter
不适用于DispatcherType.ERROR
和DispatcherType.ASYNC
.
我们可以配置 Spring Security,以使用shouldFilterAllDispatcherTypes
方法:
-
Java
-
Kotlin
@Bean
SecurityFilterChain web(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests((authorize) -> authorize
.shouldFilterAllDispatcherTypes(true)
.anyRequest.authenticated()
)
// ...
return http.build();
}
@Bean
open fun web(http: HttpSecurity): SecurityFilterChain {
http {
authorizeHttpRequests {
shouldFilterAllDispatcherTypes = true
authorize(anyRequest, authenticated)
}
}
return http.build()
}