Spring AOP 使用 JDK 动态代理或 CGLIB 为给定的代理创建代理
目标对象。JDK 动态代理内置于 JDK 中,而 CGLIB 是常见的
开源类定义库(重新打包成 )。spring-core
如果要代理的目标对象至少实现一个接口,则 JDK 动态 使用代理。目标类型实现的所有接口都是代理的。 如果目标对象未实现任何接口,则会创建 CGLIB 代理。
如果要强制使用 CGLIB 代理(例如,代理每个方法 为目标对象定义,而不仅仅是由其接口实现的对象), 你可以这样做。但是,您应该考虑以下问题:
-
使用 CGLIB,无法建议方法,因为它们不能在 运行时生成的子类。
final
-
从 Spring 4.0 开始,代理对象的构造函数不再被调用两次, 因为 CGLIB 代理实例是通过 Objenesis 创建的。仅当 JVM 执行 不允许绕过构造函数,您可能会看到双重调用和 来自 Spring 的 AOP 支持的相应调试日志条目。
-
您的 CGLIB 代理使用可能会面临 JDK 9+ 平台模块系统的限制。 典型情况下,在模块路径上部署时,无法从包中为类创建 CGLIB 代理。这种情况需要 JVM 引导标志,该标志不适用于模块。
java.lang
--add-opens=java.base/java.lang=ALL-UNNAMED
若要强制使用 CGLIB 代理,请设置属性的值
元素设置为 true,如下所示:proxy-target-class
<aop:config>
<aop:config proxy-target-class="true">
<!-- other beans defined here... -->
</aop:config>
若要在使用 @AspectJ 自动代理支持时强制执行 CGLIB 代理,请将元素的属性设置为 ,
如下:proxy-target-class
<aop:aspectj-autoproxy>
true
<aop:aspectj-autoproxy proxy-target-class="true"/>
多个部分被折叠成一个统一的自动代理创建器
在运行时,它应用任何部分(通常来自不同的 XML Bean 定义文件)指定的最强代理设置。
这也适用于 and 元素。 需要明确的是,使用 on 、 或 元素会强制使用 CGLIB
他们三个的代理。 |
多个部分被折叠成一个统一的自动代理创建器
在运行时,它应用任何部分(通常来自不同的 XML Bean 定义文件)指定的最强代理设置。
这也适用于 and 元素。 需要明确的是,使用 on 、 或 元素会强制使用 CGLIB
他们三个的代理。 |
了解 AOP 代理
Spring AOP 是基于代理的。掌握 在你写下你自己的方面或使用任何 Spring 框架提供的基于 Spring AOP 的方面。
首先考虑一个场景,你有一个普通的、未代理的、 没什么特别的,直接对象引用,如下所示 代码片段显示:
-
Java
-
Kotlin
public class SimplePojo implements Pojo {
public void foo() {
// this next method invocation is a direct call on the 'this' reference
this.bar();
}
public void bar() {
// some logic...
}
}
class SimplePojo : Pojo {
fun foo() {
// this next method invocation is a direct call on the 'this' reference
this.bar()
}
fun bar() {
// some logic...
}
}
如果在对象引用上调用方法,则该方法将直接在 该对象引用,如下图和列表所示:
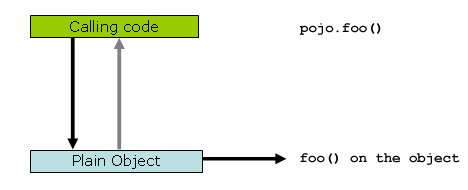
-
Java
-
Kotlin
public class Main {
public static void main(String[] args) {
Pojo pojo = new SimplePojo();
// this is a direct method call on the 'pojo' reference
pojo.foo();
}
}
fun main() {
val pojo = SimplePojo()
// this is a direct method call on the 'pojo' reference
pojo.foo()
}
当客户端代码的引用是代理时,情况会略有变化。考虑 下图和代码片段:
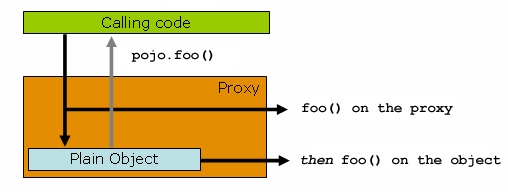
-
Java
-
Kotlin
public class Main {
public static void main(String[] args) {
ProxyFactory factory = new ProxyFactory(new SimplePojo());
factory.addInterface(Pojo.class);
factory.addAdvice(new RetryAdvice());
Pojo pojo = (Pojo) factory.getProxy();
// this is a method call on the proxy!
pojo.foo();
}
}
fun main() {
val factory = ProxyFactory(SimplePojo())
factory.addInterface(Pojo::class.java)
factory.addAdvice(RetryAdvice())
val pojo = factory.proxy as Pojo
// this is a method call on the proxy!
pojo.foo()
}
这里要理解的关键是,方法内部的客户端代码
的类具有对代理的引用。这意味着该方法调用它
对象引用是对代理的调用。因此,代理可以委托给所有
与该特定方法调用相关的拦截器(建议)。然而
一旦调用最终到达目标对象(引用
在这种情况下),它可能对自身进行的任何方法调用(例如 或 )都将针对引用而不是代理调用。
这具有重要意义。这意味着不会产生自我调用的结果
在与方法调用相关的建议中,有机会运行。main(..)
Main
SimplePojo
this.bar()
this.foo()
this
好的,那么该怎么做呢?最佳方法(使用术语“最佳” 这里松散地)是重构你的代码,这样自我调用就不会发生。 这确实需要您做一些工作,但这是最好的、侵入性最小的方法。 接下来的方法绝对是可怕的,我们犹豫不决地指出它,确切地说 因为它太可怕了。你可以(对我们来说很痛苦)完全把逻辑联系起来 在您的类中到 Spring AOP,如以下示例所示:
-
Java
-
Kotlin
public class SimplePojo implements Pojo {
public void foo() {
// this works, but... gah!
((Pojo) AopContext.currentProxy()).bar();
}
public void bar() {
// some logic...
}
}
class SimplePojo : Pojo {
fun foo() {
// this works, but... gah!
(AopContext.currentProxy() as Pojo).bar()
}
fun bar() {
// some logic...
}
}
这完全将您的代码与 Spring AOP 耦合在一起,并使类本身意识到 事实上,它被用于 AOP 上下文,这与 AOP 背道而驰。它 在创建代理时还需要一些额外的配置,因为 以下示例显示:
-
Java
-
Kotlin
public class Main {
public static void main(String[] args) {
ProxyFactory factory = new ProxyFactory(new SimplePojo());
factory.addInterface(Pojo.class);
factory.addAdvice(new RetryAdvice());
factory.setExposeProxy(true);
Pojo pojo = (Pojo) factory.getProxy();
// this is a method call on the proxy!
pojo.foo();
}
}
fun main() {
val factory = ProxyFactory(SimplePojo())
factory.addInterface(Pojo::class.java)
factory.addAdvice(RetryAdvice())
factory.isExposeProxy = true
val pojo = factory.proxy as Pojo
// this is a method call on the proxy!
pojo.foo()
}
最后,必须注意的是,AspectJ 没有这个自调用问题,因为 它不是一个基于代理的 AOP 框架。